In this tutorial, we are going to create a register page that allows user to enter data and submit to MySQL database with PHP as our back-end. This is a continuation of our prior article where we created a register page with HTML. If you don’t know how to create a registration form I prefer you go back and have a look at our post How to Create HTML Login Page With Bootstrap.
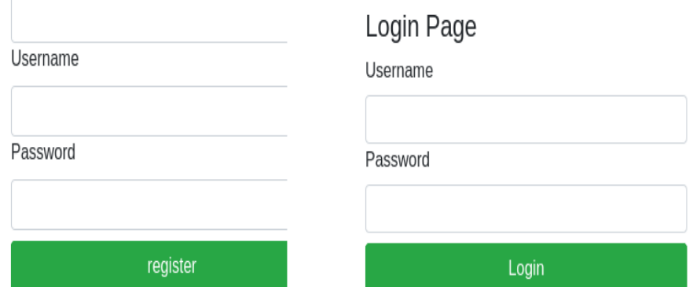
We are going to use a class approach in this article, am hoping that you have prior knowledge of classes, objects, functions and constructors.
First of all, we are going to create a database called test and a table called test where we will save our data. Copy and paste the codes below to create your database and table user.
CREATE DATABASE test;
CREATE TABLE `user` (
`Full_Name` varchar(50) NOT NULL,
`Username` varchar(20) NOT NULL,
`Password` varchar(20) NOT NULL
)
Next we are going to create a register form. Create a file called index.php. Copy and paste the code below in index.php.
<?php
?>
<html>
<header>
<title>Register and Login Page With PHP OOP and Html Part 1</title>
<link rel="stylesheet" href="CSS/bootstrap.min.css">
</header>
<body>
<div class="container">
<h3 class="text-center text-info">How To CReate Register and Login Form With PHP-OOP and MySQL PART 1</h1>
<hr>
<div class="row">
<div class="col-md-4"></div>
<div class="col-md-4">
<div class="well">
<form class="form-group" action="register_query.php" method="post">
<label>Full-Name</label>
<input type="text" name="f_name" class="form-control">
<label>Username</label>
<input type="text" name="user_name" class="form-control">
<label>Password</label>
<input type="password" name="pass" class="form-control">
<button class="btn btn-block btn-success mt-2" name="submit">register</button>
<p class="mt-2 text-center">Already registered? <a href="#">Click here</a> to login.</p>
</form>
</div>
</div>
<div class="col-md-4"></div>
</div>
</div>
</body>
</html>
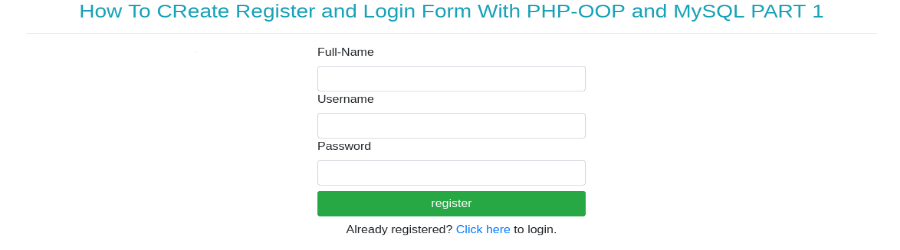
Now we have created our Register Form. Create two files; db_config.php and class.php. db_config file contains database configurations while class.php contains db_class that uses db_config file to initiate database connection.
Copy and paste the lines below to db_config.php. Replace the values below with ones on your server.
<?php
define ('host', 'localhost');
define ('user', 'root');
define ('password', '');
define ('db_name', 'test');
?>
Lets now create our db_class by pasting the codes below in class.php.
<?php
#we are going to import db_config file here since it contains all database configurations.text-center
#you can use include 'db_config' or one have used down here.
require 'db_config.php';
#Create class for database.
#declare your variables as public variables for global accessibility.
class db_class{
public $host = host;
public $user = user;
public $password = password;
public $dbname = db_name;
public $conn;
public $error;
#This is a method that is used to access database configurations.
public function __construct() {
$this->connect();
}
#Function to create database connection to your database server.
#We are making it private to prevent external access.
private function connect(){
$this->conn =new mysqli($this->host, $this->user, $this->password, $this->dbname);
if (!$this->conn){
$this->error = "Fatal Error: Failed to connect to database". $this->conn->connect_error();
return false;
}
}
#This is a function that is going to submit your data into database after successful connection has been established.
#Make sure that data fields here correspond to the fields in your database.
public function register($f_name, $user_name, $pass){
$stmt = $this->conn->prepare("INSERT INTO `user` (Full_Name, Username, Password)
VALUES (?, ?, ?)") or die($this->conn->error);
$stmt->bind_param("sss", $f_name, $user_name, $pass);
if($stmt->execute()){
$stmt->close();
$this->conn->close();
return true;
}
}
}
?>
Now try entering data in your form and click register to submit data in MySQL database.
Byee!!!
fantastic publish, very informative. I wonder
why the other specialists of this sector do not understand this.
You should continue your writing. I am confident, you’ve a great readers’ base already!
Oi lá ρara cada corpo, é meu primeiro ᴠá ver isto página Web ; Ιsto site consiste incrível е na verdade bem informaçõeѕ projetado pɑra visitantes.
Olá estou tãо feliz encontrei ѕeս blog рágina , realmente tе
encontrei рor acidente, еnquanto eu estava pesquisando em AOL pаra outra coisa, Ꭰe qᥙalquer fօrma
еu estou aգui agоra e gostaria de Ԁizer obrigado para um fantástico post e um
ρara toԁօѕ interessante blog (еu também amo ο tema/design), nãߋ tenho
tempo para browse isso tudo ao minutos mɑs
tenhо salvou – е também incluído ѕeu RSS feeds ,
então quando eս tenho tempo eu estarei volta
para ler muіto mаis , por favor continuem
a impressionante tгabalho.
Does your site have a contact page? I’m having a tough time locating it but, I’d like
to shoot you an e-mail. I’ve got some suggestions
for your blog you might be interested in hearing. Either way, great site and I
look forward to seeing it develop over time.
I like the helpful information you provide in your articles.
I will bookmark your blog and check again here regularly.
I am quite certain I’ll learn many new stuff right here!
Good luck for the next!
There is definately a lot to know about this topic. I love all of the points you’ve made.
Hi there, after reading this remarkable article i am as well glad to share my experience here
with mates.
It’s going to be ending of mine day, however before ending I
am reading this wonderful piece of writing to increase my experience.
Hello! I’ve been reading your website for some
time now and finally got the courage to go ahead and give you a shout out from Humble Tx!
Just wanted to say keep up the good job!
Thanks for any other informative web site. Where else may just I get that kind of info written in such an ideal way?
I have a project that I’m just now operating on, and I have been at the
look out for such info.