In my past tutorial, Register and Login Page With PHP-OOP and MySQL | Part 1, we only managed to create a page that allow user to register. this is by entering data in a form then submit to SQL database in server. By any chance you missed kindly refer to the link above before you continue.
For this guide, am going to create a login page with PHP OOP approach. Am going to use database I created while creating registration page. This is a continuation of the previous. To avoid confusion, please go back and check on previous work first.
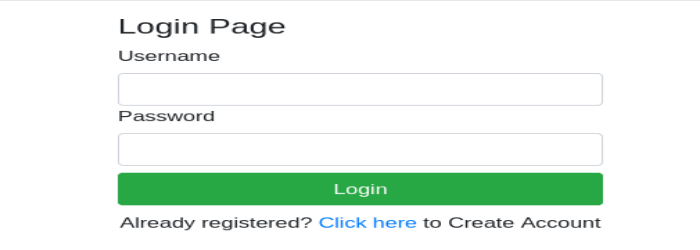
Now, let us create our login page . Copy and paste the codes below in your a file called login.php.
<?php
?>
<html>
<header>
<title> Login Page</title>
<link rel="stylesheet" href="CSS/bootstrap.min.css">
</header>
<body>
<div class="container">
<h3 class="text-center text-info">How to Create Register and Login Page With PHP-OOP and MySQL | Part 2.</h1>
<hr>
<div class="row">
<div class="col-md-4"></div>
<div class="col-md-4">
<div class="well">
<h4>Login Page</div>
<form class="form-group" action="signin.php" method="post">
<label>Username</label>
<input type="text" name="user_name" class="form-control">
<label>Password</label>
<input type="password" name="pass" class="form-control">
<button class="btn btn-block btn-success mt-2" name="login">Login</button>
<p class="mt-2 text-center">Already registered? <a href="index.php">Click here</a> to Create Account</p>
</form>
</div>
</div>
<div class="col-md-4"></div>
</div>
</div>
</body>
</html>
Code above will produce the output as shown below.

In class.php, lets create a function called public function login that will contain sql codes to fetch credentials from database.
<?php
#we are going to import db_config file here since it contains all database configurations.text-center
#you can use include 'db_config' or one have used down here.
require 'db_config.php';
#Create class for database.
#declare your variables as public variables for global accessibility.
class db_class{
public $host = host;
public $user = user;
public $password = password;
public $dbname = db_name;
public $conn;
public $error;
#This is a method that is used to access database configurations.
public function __construct() {
$this->connect();
}
#Function to create database connection to your database server.
#We are making it private to prevent external access.
private function connect(){
$this->conn =new mysqli($this->host, $this->user, $this->password, $this->dbname);
if (!$this->conn){
$this->error = "Fatal Error: Failed to connect to database". $this->conn->connect_error();
return false;
}
}
#This is a function that is going to submit your data into database after successful connection has been established.
#Make sure that data fields here correspond to the fields in your database.
public function register($f_name, $user_name, $pass){
$stmt = $this->conn->prepare("INSERT INTO `user` (Full_Name, Username, Password)
VALUES (?, ?, ?)") or die($this->conn->error);
$stmt->bind_param("sss", $f_name, $user_name, $pass);
if($stmt->execute()){
$stmt->close();
$this->conn->close();
return true;
}
}
public function login($user_name, $pass){
$stmt = $this->conn->prepare("SELECT * FROM `user` WHERE `Username` = '$user_name' && `Password` = '$pass'");
if($stmt->execute()){
$result= $stmt->get_result();
$valid = $result->num_rows;
$fetch = $result->fetch_array();
return array(
'Username' => $fetch['Username'],
'count' => $valid
);
}
}
}
?>
Next, we are going to configure a Common Gateway Interface (CGI). This is a file that is going to collect user credentials once login button is clicked and patch to class.php. Will also receive a response from public function login to facilitate login process.
create a file called login_request.php then paste the codes below.
<?php
require_once 'class.php';
if(ISSET($_POST['login'])){
$conn = new db_class();
$user_name = $_POST['user_name'];
$pass= $_POST['pass'];
$get_user = $conn->login($user_name, $pass);
if($get_user['count'] > 0){
session_start();
$_SESSION['id'] = $get_user['Username'];
echo '<script>window.location = "home.php"</script>';
}else{
echo '<script>alert("Invalid username or password")</script>';
echo '<script>window.location = "login.php"</script>';
}
}
?>
Now open login page in browser, try to login by entering valid credentials. You should be able to see the page below on your screen.
Up to this point, we have our login page working correctly. Hope you enjoyed. Thank you.
Wish you all the best in your coding career. Byee!!